Crasheye NDK for Android
Create A New App and Get The AppKey
Once you register and log in Crasheye, you can create your own APP. Every app has an exclusive AppKey to identify itself, and the AppKey will be used in initializing SDK. (Sample Download Sample )
Create new app:1. Log in Crasheye to access admin panel. 2. Click the profile picture at the upper right corner of the page, and click [my apps] in the popup menu to access app list page. 3. Click [new] in app list page. 4. Input an app name and choose a platform type, and then click [yes]. A new app is created.
Keep your unique AppKey secured from misuse.
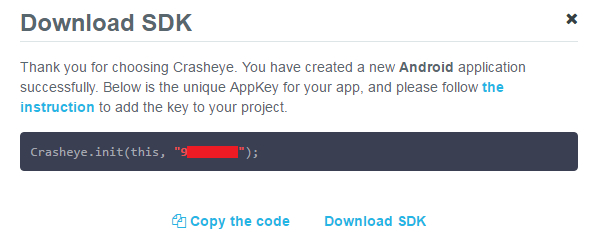
Download NDK
Click NDK dynamic library in SDK download page.
Import Dependent File
Add the downloaded Android SDK dependent file [Crasheye.jar] into [libs] folder in your project. Please refer to Add Crasheye.jar for detailed instructions.
Create [prebuild] in your project [jni] directory folder will be downloaded [libCrasheyeNDK.so]files are copied to a folder under the file.
Configuration
Configure permission settings (non-optional) and channel information (optional) in AndroidManifest.xml. Sample code is as follows:
<!--Configure permission settings, non-optional>
<uses-permission android:name="android.permission.INTERNET"/>
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE"/>
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE"/>
<uses-permission android:name="android.permission.READ_PHONE_STATE"/>
<uses-permission android:name="android.permission.READ_LOGS" />
<application ……>
<activity ……/>
<!--channel information, optional>
<meta-data android:name="CRASHEYE_CHANNEL" android:value="Your_Channel"/>
</application>
Initialization
1. Add the following code in Android.mk
########################## So loading file ##########################
include $(CLEAR_VARS)Android.mk
LOCAL_SRC_FILES := prebuild/libCrasheyeNDK.so
include $(PREBUILT_SHARED_LIBRARY)
########################## So loading file ##########################
2. Add the following code in the entry function Application.onCreate() or MainActivity.onCreate(): (Also import com.xsj.crasheye.Crasheye)
@override
public void onCreate(Bundle savedInstanceState) {
Crasheye.initWithNativeHandle(this, "Your_AppKey");
super.onCreate(savedInstanceState);
}
Your Android app is now integrated with NDK exception catching. Start the app on your Android device, and real time data will be monitored and shown on the Crasheye admin panel.
Test
Please test on a real device. If successful, data will be shown in admin panel in real time.
If successful, LogCat will output "init success!" and "ndk creah handler init success" as follows:
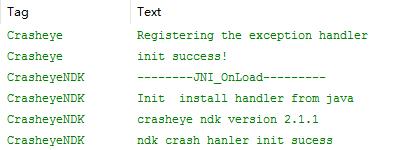
Common APIs
Channel ID
You can set Channel ID in AndroidManifest.xml; Crasheye will read the settings and report to the server. Read more: Configuration。
You can also call an API to set Channel ID without adding channel information in AndroidManifest.xml, as demonstrated in the following code sample:
@override
public void onCreate(Bundle savedInstanceState) {
Crasheye.setChannelID("Your_Channel");
Crasheye.init(this, "Your_AppKey");
super.onCreate(savedInstanceState);
}
App version
By default, Crasheye will get version information from android: android:versionName in AndroidManifest.xml. Of course you can also set app version by calling API.
@override
public void onCreate(Bundle savedInstanceState) {
Crasheye.setAppVersion("Your_APP_Version");
Crasheye.init(this, "Your_AppKey");
super.onCreate(savedInstanceState);
}
Upload via Wifi Only
In order to save the cellular data usage for the users, Crasheye offers an API to report stack trace of crashes only when the device is on wifi networks.
@override
public void onCreate(Bundle savedInstanceState) {
// true:Report via WIFI only; false: Report via whatever connected network. Default value is false.
Crasheye.setFlushOnlyOverWiFi(true);
Crasheye.init(this, "Your_AppKey");
super.onCreate(savedInstanceState);
}
User Identification
You can assign User Identification to each reported issue to help filter and locate issues. Developer can use this method to filter crash issues reported by his own device.
Crasheye.setUserIdentifier(String userIdentifier);
Log Collection
This API collects logs (LogCat) in runtime. When exception occurs, the collected logs will be saved and reported to server. (Maximum 1000 lines of the latest logs)
/**
* Set line limit and keyword to access logs
* @param lines : lines of codes collected (1000 lines in maximum)
* @param filter : keyword(require = false), for example, using Crasheye as keyword is equivalent to entering "logcat -d Crasheye" in Logcat filter
*/Crasheye.setLogging(int lines, String filter);
Breadcrumb (Navigation Information)
By leaving breadcrumbs (navigation information), you can capture the time and sequence of crashes and handled exceptions to keep track of if your app is working as intended. Call the following API where you want to monitor:
Crasheye.leaveBreadcrumb(String breadcrumb);
Developer-Defined Text String
In case that you want to capture more data than the default setup does, you can call this API to add developer-defined text string to collect more information:
Crasheye.addExtraData(String key, String value);
Report Script Exception
This API is for the convenience of developers. The caught exceptions of stack trace, such as lua and js script exception, will be reported to server. (In order to reduce repetitive reports, the same exception will only be reported once in 10 minutes)exceptions of stack trace
/**
* Script exception reporting interface
* @param errorTile : title of script exception
* @param stacktrace : stack trace
* @param language : script language
*/
Crasheye.sendScriptException(String errorTitle, String stacktrace, String language);
Callback Function for NDK Exception
When NDK exception occurs, Crasheye calls the developer-specified function back to collect key information.
/**
* Set callback function for NDK exception
* @param ndkExceiptn : implement NDKException callback interface
*/
Crasheye.setNDKExceptionCallback(NDKExceptionCallback ndkExceiptn);
Device ID
Device ID is accessible via this API. Users can filter the logs with device ID to find the matched exceptions of the device.
Crasheye.getCrasheyeUUID();
Set Beta Version
/**
* Set whether the version is the beta
* @param isBeta : true is beta version
*/
Crasheye.setIsBetaVersion(boolean isBeta)
Symbol File Upload
1. Environment
Java SE Runtime Environment 7 or later
2. Preparation
a. Symbol file upload tool
b. Debug SO file (SO file with debugging information).Location:
If IDE is Eclipse + NDK, the path of Debug SO file is by default:
<project folder>/obj/local/<framework>/
If IDE is Android + NDK, the path of Debug SO file (debug build) is by default:
<Project folder>/build/intermediates/ndk/debug/obj/local/<architecture>/
Path of Debug SO file (release build) is by default:
<Project folder>/build/intermediates/ndk/release/obj/local/<architecture>/
3.Symbol File Upload
Parameters | Meaning |
---|---|
-appkey | Appkey of the project |
[-veresion] | app version (optional), read from AndroidManifest.xml by default |
[-AndroidManifestPath] | AndroidManifest.xml file path (to get app version, being mutually exclusive with the parameter -version) (optional) |
[-disableUpload] | disable symbol file upload |
[-dumpsyms] | This is the location of dump_syms, which is a dsym analyzing system. It points to the current working directory by default. |
Via website
1. Start the upload tool and use the following command to zip the symbol file:
java -jar CrasheyeAndroidSymbol.jar -disableUpload symbol_file_path
2. Upload the newly created.zip file via website (Settings -> Symbols -> Upload Symbol File), and select a matched app version.
Via upload tool
1. Start the symbol file upload tool, and use the following command to upload:
java -jar CrasheyeAndroidSymbol.jar -appkey=project's AppKey [-version=App version] [-AndroidManifestPath=AndroidManifest.xml path]